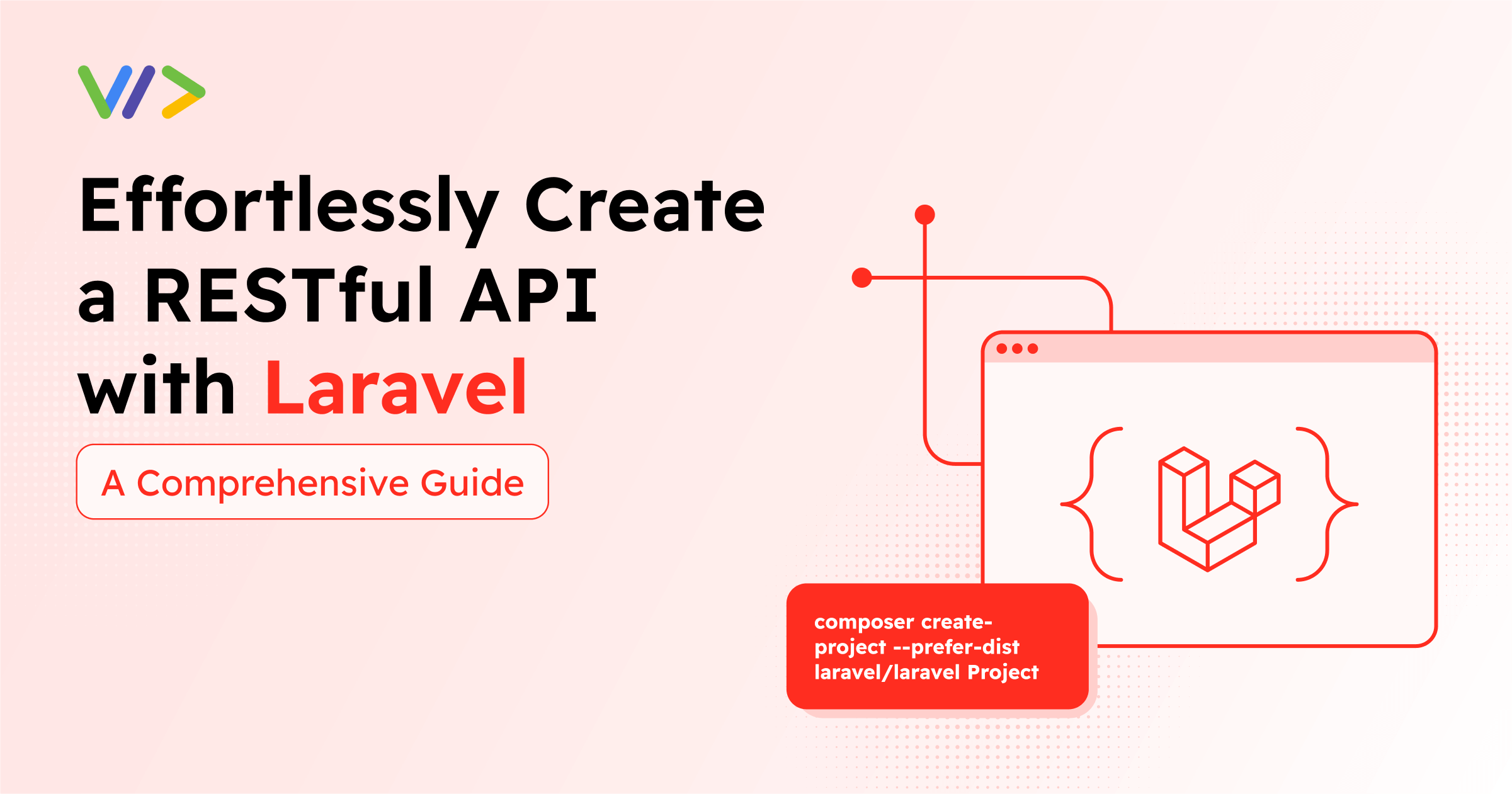
Introduction
The demand for seamless and efficient interconnectivity between various software applications has never been higher. This is where RESTful APIs come in – they act as the beating heart of today’s application ecosystem, enabling different systems to communicate with each other effortlessly. Laravel, a powerful PHP framework, is perfectly suited for building these APIs due to its elegant syntax, robust features, and the ease with which Laravel developers can get up and running with it.
In this comprehensive guide, we will walk through every step of creating a RESTful API using Laravel. Whether you are new to Laravel or have some experience, this guide aims to provide valuable insights that will help in building a robust, secure, and scalable API.
Setting Up Your Laravel Environment
Installing Laravel
Before diving into the world of Laravel, ensure your system meets the following requirements: PHP 7.3.0 or higher, Composer, and a suitable database system like MySQL or SQLite.
Here is a simplified step-by-step process to get Laravel installed on your machine:
- Open your terminal or command prompt.
- Run
composer create-project --prefer-dist laravel/laravel YourProjectName
to create a new Laravel project. - Once the installation is complete, you can run
php artisan serve
from your project directory to start Laravel’s development server.
Setting up a Development Environment
Choosing the right development environment is crucial for a productive coding experience. Laravel offers Homestead, a pre-packaged Vagrant box that provides a development environment without requiring you to install PHP, a web server, and any other server software on your local machine. Alternatively, for those who prefer a lightweight solution, Laravel Valet is a great choice for macOS users, and for those who like containerized environments, Docker can be integrated with Laravel seamlessly.
Preparing Laravel for API Development
After setting up your environment, some initial configuration steps are necessary:
- Familiarize yourself with the Laravel project structure.
- Configure necessary environment variables in the .env file.
- Set up database connections and any other services your API will use.
Building the RESTful API with Laravel
Designing the Database
Effective database design is crucial for any API. Laravel uses migrations to define your database tables and columns, and Eloquent ORM for interacting with your database.
Here are the general steps:
- Plan your database structure.
- Use Laravel migrations to create your database tables. Run
php artisan make:migration create_your_table_name_table
in the terminal. - Use Eloquent, Laravel’s ORM, to interact with your database elegantly.
Creating Models and Controllers
This step involves generating models and controllers necessary for your API. Each model in Laravel corresponds to a table in your database.
- Run
php artisan make:model YourModelName
to create a model. - Controllers can be created using “`php artisan make:controller YourControllerName.
- For APIs, consider generating resource controllers that come with methods corresponding to standard HTTP verbs.
Implementing CRUD Operations
CRUD operations form the backbone of any RESTful API, allowing users to create, read, update, and delete resources.
- GET: Fetch data using Eloquent ORM and return responses in JSON format.
- POST: Accept data through API requests and use models to insert data into the database.
- PUT/PATCH: Update resources in your database.
DELETE: Remove resources from your database.
Securing Your Laravel API
Authentication and Authorization
When it comes to securing your API, Laravel offers several options like Passport, Sanctum, and JWT for authentication.
- Choose the one that best suits your project requirements.
- Implement authentication middleware to protect your routes.
- Use policies and gates to authorize user actions within your API.
Validating Requests
Laravel provides a powerful validation system:
- Use form requests to validate incoming API requests.
- Customize validation rules as per your needs.
- Properly handle validation errors and provide meaningful error responses.
Protecting Against Common Security Threats
- Use Laravel’s built-in protections against SQL injection.
- Set up CORS to secure your API from cross-origin requests.
- Implement SSL/TLS encryption to protect data in transit.
Testing and Documentation
Writing API Tests
Testing is an integral part of API development. Laravel’s built-in testing capabilities like feature tests are powerful tools for ensuring your API works as intended.
Documenting the API
Clear documentation is essential for any API. Use tools like Swagger or API Blueprint to document your Laravel API. This aids in maintaining clarity and ensuring ease of use for developers integrating with your API.
Versioning Your API
API versioning is crucial for maintaining backward compatibility while introducing new features. There are several strategies you can adopt for versioning your API in Laravel.
Optimizing and Scaling Your Laravel API
Best Practices for Performance Optimization
- Index your database tables.
- Implement caching strategies.
- Use eager loading to reduce the number of database queries.
Scaling Your Laravel Application
Understand when to scale horizontally (adding more machines) or vertically (adding resources to existing machines). Implement load balancing to distribute traffic evenly.
Conclusion and FAQs
Building a RESTful API with Laravel is an enriching journey that combines the elegance of Laravel with the robustness required for modern API development. Through careful planning, coding, testing, and documentation, you can create APIs that are not only functional but also secure, scalable, and easy to use.
FAQs
- How do I choose the right authentication method for my API?
Consider your project requirements, the level of security needed, and your familiarity with the technology. - What are the best practices for API versioning?
Consistency is key. Decide on a versioning strategy early and stick to it. - How can I monitor the performance of my Laravel API?
Use Laravel’s built-in capabilities along with external tools like New Relic or Prometheus. - When should I consider scaling my Laravel application?
Monitor your application’s performance, and look for signs of strain under load, which could indicate it’s time to scale.
By approaching each step of this guide with a clear plan and a focus on best practices, you can build a RESTful API that stands the test of time.